What is GraphQL?
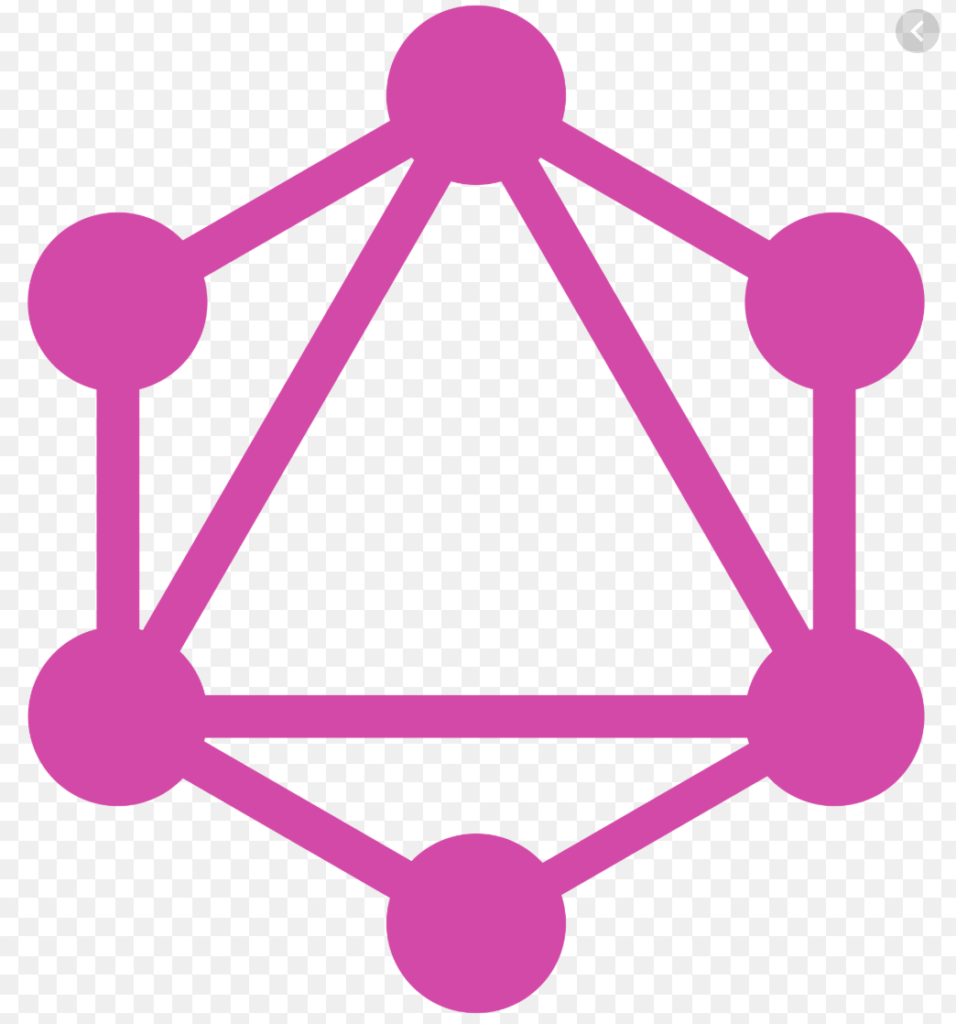
GraphQL solves the problem of writing Declarative, Compositional and Strongly Typed HTTP handler(s). The purpose of GraphQL is to make it easy to query complex data about multiple entities. GraphQL enables you to fetch in one request, which would take multiple requests in REST.
Issues with REST:
- Poor performance
- A lot of endpoints
- Over-fetching or under-fetching of data
- Difficulty understanding APIs
- Difficulty API versioning
Basically, GraphQL provides a structure of how to handle client requests (for querying or mutating data).
To really understand what it does let’s go over what you need to do when writing an API handler to provide some data to clients without GraphQL.
Let’s write an HTTP API handler for your Profile info, In node or in any other backend language you will write –
- A GET request with some routing like /get/profile/info/:userId
- You’ll write some code to return data on that route (which will fetch data from the DB), and you will return data something like =
For example:
{
“response”: {
“name”: “Rob”,
“age”: 45
}
}
- Now if front end developer wan to use this API, they need whole API Routing and Structuring Info, either they will discuss it with back end developer or will go through the back end API code.
Now, what If you want to use this User info as well as his other info like user activity as well?
Now there are several ways to solve this:
- We can add another route: /get/profile/activity/:userId and go through the process described above again. The client will make 2 calls to both routes and he’ll have both information
- Allow customizing the profile result. If you’ve been developing for a while this will probably look familiar /get/profile/activity/:userId?with_activity=true.
There’s no standard or a good rule of thumb on which way to go. As the project grows it becomes increasingly hard to manage the number of routes and the scope and structure of what each of them returns. There’s no good way to do it other than browsing the code and see what is returned.
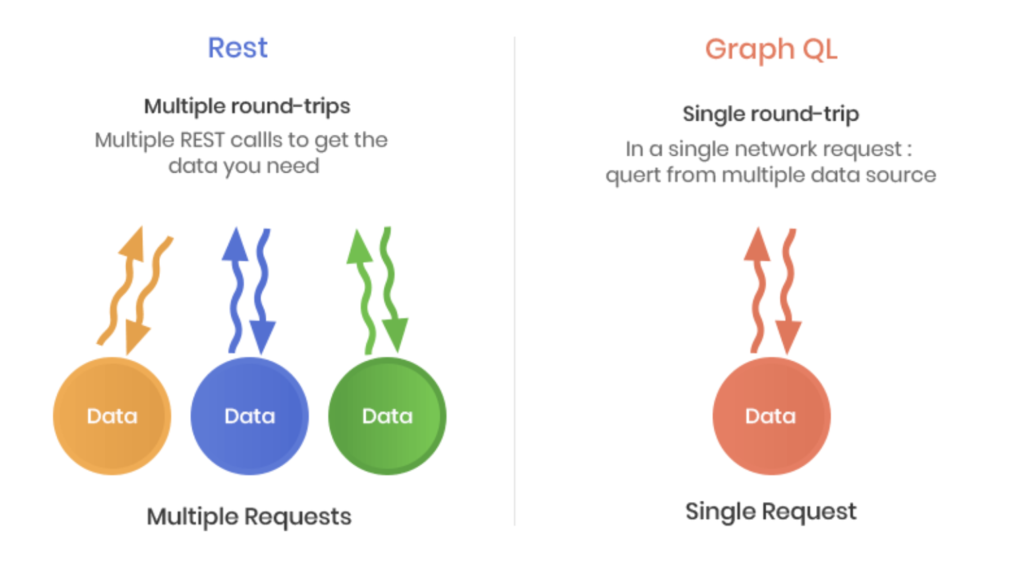
This is where GraphQL comes in:
- There are no routes.
You just need a single route (ex: /graphql) that can take a graphql query as input and return the result - There’s a schema — so you can look and see all the queries available and what kind of data they return
- You know how the result looks like simply by looking at the query!
{
user(id:1234) {
id,
name,
age,
activity
}
}
returns
{
“user” : {
“id”: 1234,
“name”: “Rob”,
"age": 45
"activity" : {
browse: true
}
}
}
So client developers can quickly see what data is available to them, make a query and get a result they understand without having to go through the server’s HTTP code.
What GraphQL provides us :
- Minimize data fetching
- Minimize Application endpoint
- Optimize API Versioning
Conclusion
GraphQL might seem complex at first because it’s a technology that reaches across many areas of modern development. But if you take the time to understand the underlying concepts, I think you’ll find out that a lot of it just makes sense.