Practical Use of debounce and throttle in web development
I am sure as a web developer you must have heard about buzz word Debounce and Throttling. These are very common techniques to improve your web performance. Have ever wonder how google, amazon search suggestions work so seamlessly ?, Yes these techniques are behind the scene to provide you these experiences. So let’s begin.
Theoretically, they are –
Throttling enforces a maximum number of times a function can be called over time. As in “execute this function at most once every 100 milliseconds.”
Debouncing enforces that a function will not be called again until a certain amount of time has passed without it being called. As in “execute this function only if 100 milliseconds have passed without it being called.”
All good, Right ! So, here in this article we will discuss some use cases where it makes sense to use them.
Case Study 1: In this case we will try to build an efficient search box for an ecommerce website. Like Amazon Search.
We have an input box where user will type some words ( letters) and as per the data we will make search API call to show suggestion data. Generally we bind the keypress event of the input box to fire the search API, and after successful execution we show result in drop box.
Something fishy ? No, Ok –
Notice that each time the keypress event fires, it makes API call and renders the response data on the screen. In fact, those results haven’t been seen by the user, because they have been overridden by the subsequent results of the latest keypress event. Right. It’s very bad user experience to skip or show wrong data to the user. So how we can solve this issue ?
What if we delay (after last keypress) our search API call by 500ms ?
We will make our search API call only after user finishes typing (500ms delay). We are avoiding many unnecessary API calls by doing so. Also it will reduce code rendering as well.
This is called debouncing, where we are enforcing that a function (API) will not be called again until a certain amount of time has passed. Here is snippet how we can write util to debounce our function.
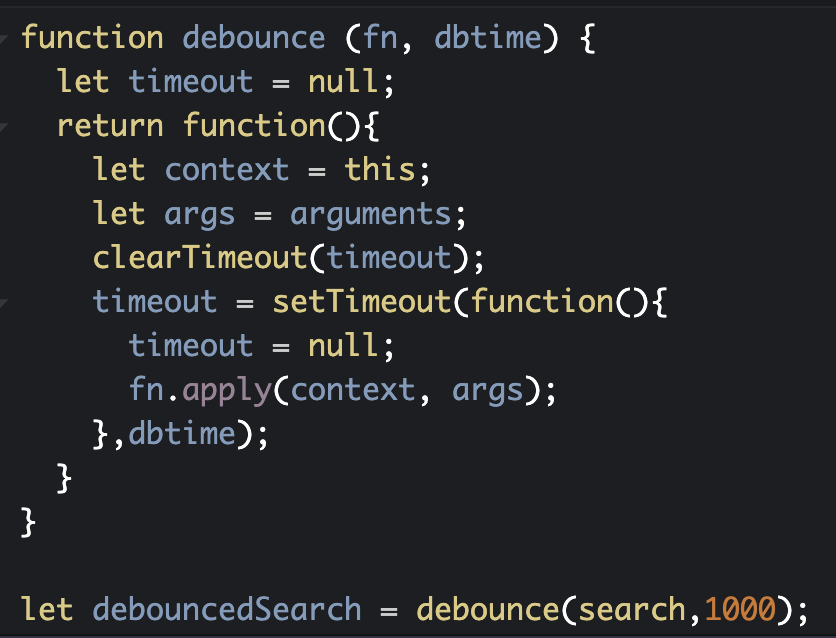
This is all about Debounce.
Case Study 2 : “infinite scroll” in a web page.
In this case, we calculate the difference between the end of the page and the current page position and loads more content ( Making API call and loading more data ) if this difference is small enough. Here is a visual representation of what I am saying.
If we write our code in simple manner using JQuery it will look like something –
$(window).on(‘scroll’, function() {
if (window.pageYOffset > loadMoreButton.offsetTop – 1000)
# load more content via ajax
}
now, the problem is that every time we scroll, scroll event fires multiple times per scroll. But we don’t want this, we want to call our API once when we reach at that point, Right ?
So what we can do ?
One thing we can define a time interval and only process a scroll event once within that time interval, If more than one scroll event comes in during that time interval, you ignore it and process it only when that time interval has passed.
This is called throttling, we just throttled our infinite scroll call to solve this issue. Hefe we are enforcing maximum number of times a function can be called over time.
Here is the sudo code lib use to create throttle function-
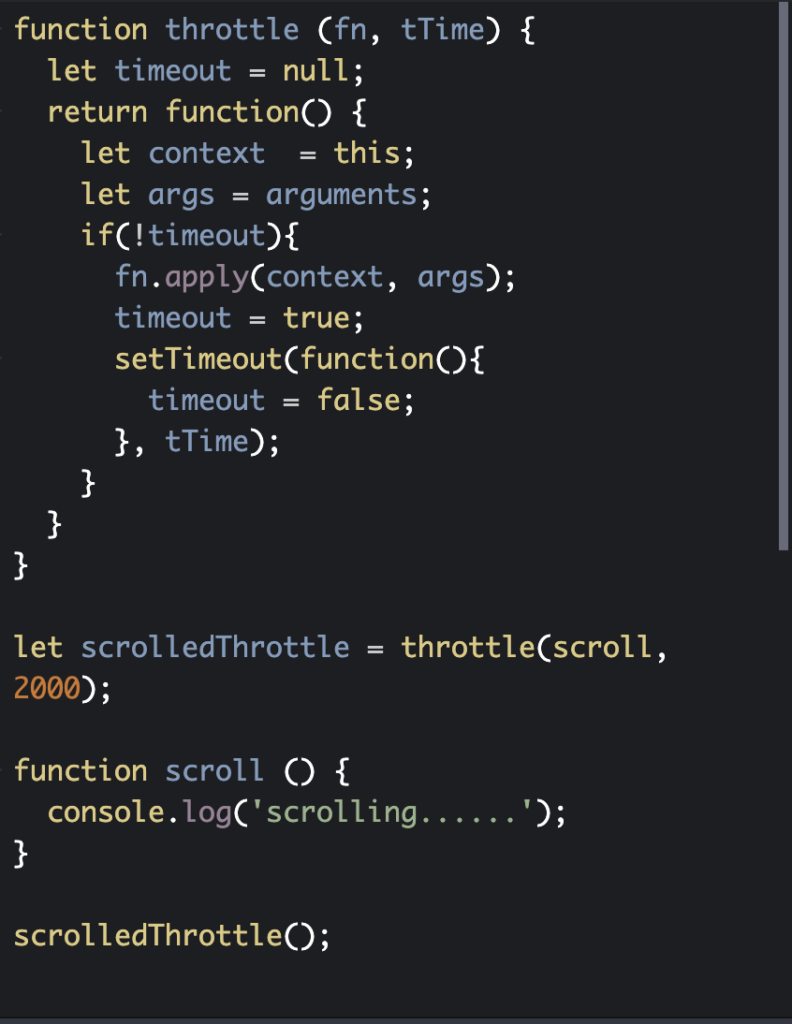
That’s all on throttling.
Conclusion – Throttling and Debounce are very effective processes which we can use to improve our user experience. As you can see in both examples performance and user experience is very important and Debouncing and Throttling are pinpointed solutions for both of them.